BIOS Video Services
BIOS video services, accessible via interrupt INT 0x10
, provide a range of functions to interact with the video hardware in real mode. These services allow you to set video modes, manipulate the screen, and manage video memory, which is crucial for developing bootloaders and low-level system software.
Here's an overview of the key BIOS video services:
Key BIOS Video Services
[ 1 ] Set Video Mode (INT 0x10, AH = 0x00
)
- Purpose: Set the display to be a specific mode.
- Different video modes will be explained later in this chapter.
- Usage: (Setting mode 3)
mov ah, 0x00 ; Function: Set Video Mode
mov al, 0x03 ; Mode: 3 (80x25 text)
int 0x10 ; Call BIOS video interrupt
[ 2 ] Set Cursor Position (INT 0x10, AH = 0x02
)
- Purpose: Move the cursor to a specific position on the screen.
- Usage:
mov ah, 0x02 ; Function: Set Cursor Position
mov bh, 0x00 ; Page number (usually 0)
mov dh, 0x0A ; Row (Y coordinate)
mov dl, 0x05 ; Column (X coordinate)
int 0x10 ; Call BIOS video interrupt
[ 3 ] Get Cursor Position (INT 0x10, AH = 0x03
)
- Purpose: Retrieve the current cursor position and shape.
- Usage:
mov ah, 0x03 ; Function: Get Cursor Position
mov bh, 0x00 ; Page number (usually 0)
int 0x10 ; Call BIOS video interrupt
; Cursor position returned in DH (row) and DL (column)
[ 4 ] Write character and Attribute at Cursor (INT 0x10, AH = 0x09
)
- Purpose: Write a character at the current cursor position with a specified attribute without advancing the cursor.
- Typical Usage: Drawing characters with specific attributes at fixed position on the screen.
- Parameters:
AH = 0x09
: Function number for writing character and attribute.AL
: ASCII value of the character to write.BH
: Page number (usually 0).BL
: Attribute byte (specifies foreground and background colors).CX
: Number of times to write the character.
mov ah, 0x09 ; Function: Write Character and Attribute
mov al, 'A' ; Character to write
mov bl, 0x0F ; Attribute (white on black)
mov cx, 1 ; Number of times to write the character
int 0x10 ; Call BIOS video interrupt
Characteristics:
- Does not automatically advance the cursor position.
- Useful for setting a block of characters with specific attributes.
- Allows writing the same character multiple times by setting
CX
.
[ 5 ] Teletype Output (INT 0x10, AH = 0x0E
)
- Purpose Writes a character at the current cursor position and advances the cursor to the next position.
- Typical Usage: Displaying text, where the cursor moves automatically after each character.
- Parameters:
AH = 0x0E
: Function number for teletype output.AL
: ASCII value of the character to write.BH
: Page number (usually 0).BL
: Text attribute (only used in graphics mode; ignored in text mode).
mov ah, 0x0E ; Function: Teletype Output
mov al, 'A' ; Character to write
mov bh, 0x00 ; Page number (usually 0)
mov bl, 0x07 ; Attribute (white on black), ignored in text mode
int 0x10 ; Call BIOS video interrupt
Characteristics:
- Advances the cursor position automatically after writing the character.
- Useful for sequential text output.
- The attribute byte in
BL
is only relevant in graphics modes; in text modes, the current attribute setting is used.
[ 6 ] Scroll Screen (INT 0x10, AH = 0x06 and AH = 0x07
)
Scroll Screen Down, (INT 0x10, AH = 0x06)
- Purpose: Scrolls the screen up by a specified number of lines.
- Parameters:
AL
: Number of lines to scroll up.BH
: Attribute for blank lines (graphics mode only).CH
,CL
: Row and column of the upper-left corner of the scroll rectangle.DH
,DL
: Row and column of the lower-right corner of the scroll rectangle.
mov ah, 0x06 ; Function: Scroll Screen Up
mov al, 1 ; Number of lines to scroll up
mov bh, 0x00 ; Attribute for blank lines (ignored in text mode)
mov cx, 0 ; Upper-left corner (row, column)
mov dx, 0x184F ; Lower-right corner (row, column)
int 0x10 ; Call BIOS video interrupt
Scroll Screen Down (INT 0x10, AH = 0x07)
- Purpose: Scrolls the screen down by a specified number of lines.
- Parameters:
AL
: Number of lines to scroll down.BH
: Attribute for blank lines (graphics mode only).CH
,CL
: Row and column of the upper-left corner of the scroll rectangle.DH
,DL
: Row and column of the lower-right corner of the scroll rectangle.
mov ah, 0x07 ; Function: Scroll Screen Down
mov al, 1 ; Number of lines to scroll down
mov bh, 0x00 ; Attribute for blank lines (ignored in text mode)
mov cx, 0 ; Upper-left corner (row, column)
mov dx, 0x184F ; Lower-right corner (row, column)
int 0x10 ; Call BIOS video interrupt
[ 7 ] Checking Video Mode (INT 0x10, AH = 0x0F
)
- Purpose: To check the video mode.
- Parameters:
AH
: 0x0F
- Return:
- AL: video mode.
; Get current video mode
mov ah, 0x0F ; Function: Get current video mode
int 0x10 ; Call BIOS video interrupt
; Video mode is returned in AL
Understanding Video Modes in BIOS
BIOS provides a set of functions to interface with the hardware, including video display settings. These functions are accessible through interrupt calls, specifically INT 0x10
, which handles video services. Video modes can range from simple text modes to complex graphics modes, depending on the requirements of your bootloader.
- In BIOS real mode, video modes are divided into text modes and graphics modes.
- Each mode has a specific characteristics regarding resolution, color depth, and memory usage.
- These modes range from text modes to various graphic modes, each with different resolutions and color depths.
Here's an overview of some commonly used video modes in real mode:
Text Modes:
Text modes are used for displaying characters on the screen. They offer various resolutions and color depths.
- Text Mode memory starts from
0xB8000
[1] 80x25 Text Mode (Mode 3)
- This is the Default Video Mode in most BIOS's.
- Resolution: 80 columns by 25 rows
- Color depth: 16 colors
- Memory address:
0xB8000
- Usage: Standard text display with 16 colors
[2] 40x25 Text Mode (Mode 1)
- Resolution: 40 columns by 25 rows
- Color depth: 16 colors
- Memory address:
0xB8000
- Usage: Larger text for visibility, useful in early computing
[3] 80x50 Text Mode (Mode 3h)
- Resolution: 80 columns by 50 rows
- Color depth: 16 colors
- Memory address:
0xB8000
- Usage: More text on screen for higher information density
Graphics Modes
Graphics mode are used for displaying images and graphical content. They offer various resolutions and color depths.
- Graphic Modes video memory starts from
0xA0000
[1] 320x200 Graphics Mode (Mode 13h, 256 colors)
- Resolution: 320 pixels by 200 pixels
- Color depth: 256 colors (8 bits per pixel)
- Memory address:
0xA0000
- Usage: Popular for games and simple graphics applications
[2] 640x480 Graphics Mode (Mode 12h, 16 colors)
- Resolution: 640 pixels by 480 pixels
- Color depth: 16 colors (4 bits per pixel)
- Memory address:
0xA0000
- Usage: Higher resolution graphics with limited color depth
[3] 640x200 Graphics Mode (Mode 6, 2 colors)
- Resolution: 640 pixels by 200 pixels
- Color depth: 2 colors (monochrome), 1 bit per pixel.
- Memory address:
0xA0000
- Usage: Early high-resolution graphics for simple images
[4] 320x240 Graphics Mode (Mode X)
- Resolution: 320 pixels by 240 pixels
- Color depth: 256 colors (requires tweaking Mode 13h)
- Memory address:
0xA0000
- Usage: Higher vertical resolution than Mode 13h, used in some games
Setting Video Modes
In order to set video mode, BIOS provides function 0x00
in the interrupt 10h
.
- Setting a Text Mode :-
Here is an example of setting the 80x25
text mode with 16 colors (mode 0x03
):
; Set video mode 0x03 (80x25, 16 colors text mode)
mov ah, 0x00 ; Function 0x00: Set a video mode
mov al, 0x03 ; Mode 0x03: 80x25 text mode
int 0x10 ; BIOS video service
- Setting a Graphic Video Mode :-
; Set video mode 13h (320x200, 256 colors)
mov ax, 0x0013
int 0x10
Note: Changing the video typically clear the screen. When you switch from one video mode to another using the BIOS interrupt INT 0x10, the contents of the screen are reset. This means any text or graphics displayed in the previous mode will be erased, and the screen will be initialized to a default state (usually black or a blank screen depending on the mode).
What is Linear Address?
A linear address in video memory refers to the contiguous range of memory address (mapped) used to represent the screen's pixels. Each pixel's color value is stored at a linear address, allowing the CPU to directly access and modify specific pixels.
Video Memory Layout in Mode 13h
- Resolution: 320 pixels (width) by 200 pixels (height)
- Color Depth: 256 colors (8 bits per pixel, 1 byte per pixel)
- Video Memory Base Address: 0xA0000
- Total Memory Required: 320*200 = 64,000 bytes.
Graphical Representation
Step-by-Step Graphical Breakdown
- Starting at the top-left corner (0,0):
- The offset for the top-left pixel is
0
.
- The offset for the top-left pixel is
- Moving horizontally:
- Each pixel to the right increases the offset by
1
.
- Each pixel to the right increases the offset by
- Moving vertically:
- Each new row starts after
320
pixels from the previous row, so the first pixel of the second row has an offset of320
, the first pixel of the third row has an offset of640
, and so on.
- Each new row starts after
- Combining horizontal and vertical movements:
- To find the offset for any pixel, multiply the row number (Y) by the row length (320) and add the column number (X).
Visual Representation:
Screen (320x200)
Offset calculation for pixel (100, 50)
-------------------------------------
Row 0: 0, 1, 2, ..., 99, 100, 101, ..., 319
Row 1: 320,321,322, ..., 419, 420, 421, ..., 639
...
Row 50:16000,16001,16002,...,16099,16100,16101,...16319
...
Calculating Linear Addresses
To find the linear address for a pixel at coordinates (X, Y), you need to follow these steps:
- Start with the base address of the video memory: 0xA0000
- Multiply the Y coordinate by the screen width (320) to get the row offset.
- Add the X coordinate to the row offset to get the final offset.
Mathematical Formula:
linear address = base address + (Y * screen_width) + X
where, screen_width = 320
and base address = 0xA0000
Example Calculation:
Let's calculate the linear address for a pixel at coordinates (100, 50):
- X-coordinate = 100
- Y-coordinate = 50
- Screen width = 320
- Base address =
0xA0000
Applying the formula:
linear address = 0xA0000 + (50 * 320) + 100
0xA0000 + (16100)it is in decimal
convert the decimal part to hex
0xA0000 + 0x3EE4
0xA3EE4
Example Code to Set the Video Mode
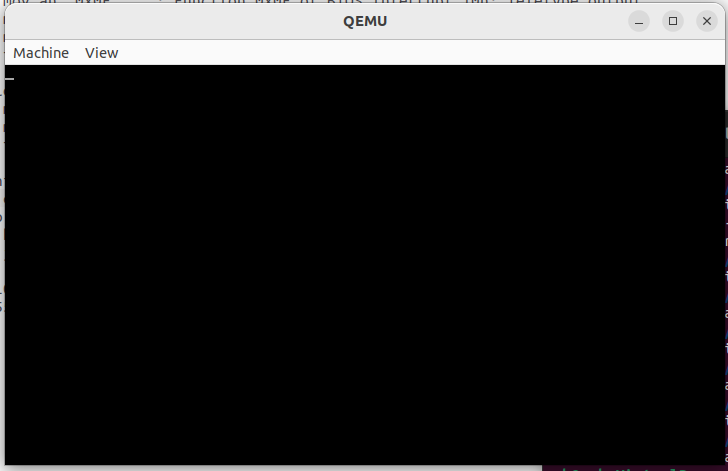