Overview
While Input properties allow data to flow from parent components to child components, output properties (often referred to as ‘Events Up’) enable child components to communicates with their parent components. In this chapter, we will explore output properties in Angular, with detailed examples and explanations for each line of code.
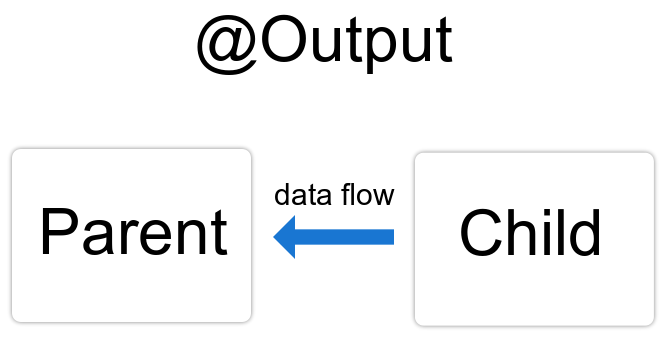
Understanding Output Properties
Output properties provide a way for child components to send data or trigger events that can be captured and handled by their parent components. This mechanism is essential for building interactive and responsive applications.
Defining Output Properties
To define an output property in a child component, you use the @Output
decorator along with an instance of the EventEmitter
class. The @Output
decorator marks a property as an output property, and the EventEmitter
is used to emit events. Here's how you can define an output property:
import { Component, EventEmitter, Output } from '@angular/core';
@Component({
selector: 'app-child',
template: `
<button (click)="emitEvent()">Click me</button>
`,
})
export class ChildComponent {
@Output() childEvent = new EventEmitter<void>();
emitEvent() {
this.childEvent.emit();
}
}
In the code above:
- We import the necessary modules and decorators, including
EventEmitter
andOutput
. - We define a child component with a button in its template.
- We declare an output property called
childEvent
with the@Output
decorator, which is an instance ofEventEmitter<void>
. This output property will emit events with no data when triggered.
Binding Output Properties
To capture the events emitted by the child component in the parent component, you can use event binding. Here's how you can do it:
<app-child (childEvent)="handleChildEvent()"></app-child>
In this code:
- We place the child component (
<app-child>
) in the parent component's template. - We use event binding
(childEvent)
to listen for events emitted by the child component and specify the methodhandleChildEvent()
to execute when the event is triggered.
Handling Output Events
In the parent component, you need to define the handleChildEvent()
method to handle the event when it's triggered. Here's an example:
import { Component } from '@angular/core';
@Component({
selector: 'app-parent',
template: `
<div>
<h1>Parent Component</h1>
<app-child (childEvent)="handleChildEvent()"></app-child>
</div>
`,
})
export class ParentComponent {
handleChildEvent() {
console.log('Event received from child component.');
// Add your event handling logic here
}
}
In the parent component code:
- We import the necessary modules.
- We define a
handleChildEvent()
method that will execute when the event is triggered. - We use event binding to capture the event emitted by the child component and call the
handleChildEvent()
method.
Leave a comment
Your email address will not be published. Required fields are marked *