Understanding Plane Geometry
Plane Geometry simplifies the creation of flat surfaces in Three.js. Think of it as the canvas upon which you can paint your digital masterpieces. The class takes parameters defining the width and height of the plane, allowing for easy customization.
// Creating a PlaneGeometry
const planeWidth = 5;
const planeHeight = 5;
const planeGeometry = new THREE.PlaneGeometry(planeWidth, planeHeight);
Materializing the Plane
To make the plane visible, it must be combined with a material to form a mesh. Materials determine how light interacts with the surface, influencing the appearance of the plane.
const SquarePlane = new THREE.PlaneGeometry(100, 100);
// Creating a textured plane mesh
const texture = new THREE.TextureLoader().load('assets/bricks-wall.jpg');
const texturedMaterial = new THREE.MeshBasicMaterial({ map: texture });
const texturedPlaneMesh = new THREE.Mesh(SquarePlane, texturedMaterial);
texturedPlaneMesh.position.set(0, 0, -100);
scene.add(texturedPlaneMesh);
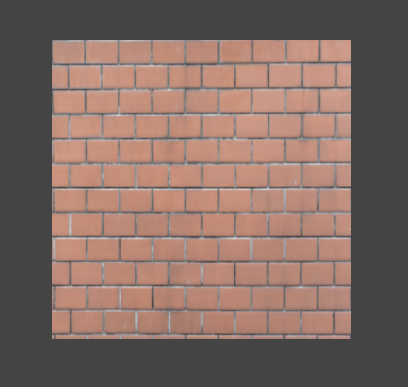
Transforming and Animation
As with other geometries in Three.js, Plane Geometry seamlessly integrates with transformations. This means developers can dynamically position, rotate, and scale the plane, adding life and movement to the scene.
// Animating the plane
function animate() {
requestAnimationFrame(animate);
texturedPlaneMesh.rotation.z += 0.01;
// or texturedPlaneMesh.rotateZ(0.01);
renderer.render(scene, camera);
}
animate();