Overview
Canvas is a HTML5 element that allows you to draw graphics and animations directly within your web pages using JavaScript. In this chapter, we will introduce the Canvas element, and explore its basic functionalities. By the end of this chapter, you will have a solid understanding of how to set up a Canvas in HTML, draw basic shapes, and interact with them using JavaScript.
Introduction to Canvas
Canvas is an HTML5 element that provides a drawable region on a web page. It allows for dynamic rendering of graphics, animations, and interactive content. Unlike SVG (Scalable Vector Graphics), which uses XML to describe 2D graphics, Canvas provides a bitmap-based drawing surface where you can draw shapes, lines, text, and images programmatically using JavaScript.
Setting up Canvas
To use Canvas, you first need to create a Canvas element in your HTML document. Here's how you can set up Canvas:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset=UTF-8>
<meta name=viewport content="width=device-width, initial-scale=1.0">
<title>Canvas Demo</title>
<style>
canvas {
border: 1px solid black;
}
</style>
</head>
<body>
<canvas id="myCanvas" width=400 height=200></canvas>
<script>
// JavaScript code will go here
</script>
</body>
</html>
Output:
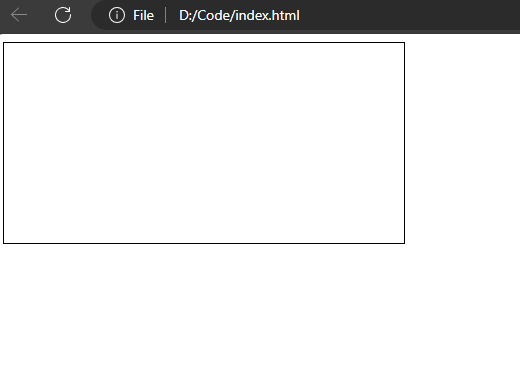
Setting the dimensions of Canvas
There are two ways to setting the dimensions of the Canvas either by HTML or through JavaScript.
In HTML we can set its dimensions with the width
and height
property, if we set them to 100% then canvas takes the entire space.
With JavaScript, we can get the Canvas element and then set the element property with the width and height property, as shown in the snippet below:
// Get the Canvas element
const canvas = document.getElementById('myCanvas');
// HardCoded dimensions
// Set the dimensions
canvas.width = 800; // Set the width to 800 pixels
canvas.height = 600; // Set the height to 600 pixels
// OR
// Set the dimensions to match the window size
canvas.width = window.innerWidth;
canvas.height = window.innerHeight;
Canvas Coordinate System
Canvas uses a Cartesian coordinate system, where the origin (0, 0) is located at the top-left corner of the Canvas element. The x-axis increases from left to right, and the y-axis increases from top to bottom, You can use this coordinate system to position and draw shapes on the Canvas.
The Canvas space doesn't have visible negative points. Using negative coordinates will position objects outside the canvas so that the objects will not appear on the page.
Canvas Context
To draw on the Canvas, you need to obtain the drawing context using JavaScript. The getContext()
method is used to retrieve the rendering context of the Canvas, typically “2d” for a 2D rendering context. Once you have the context, you can set drawing styles such as colors, line widths, and line styles using various properties and methods.
Setting Canvas Background Color
We can set the background color of the Canvas element using the style property:
let canvas = document.getElementById('myCanvas');
canvas.style.background = "#ff00ff";
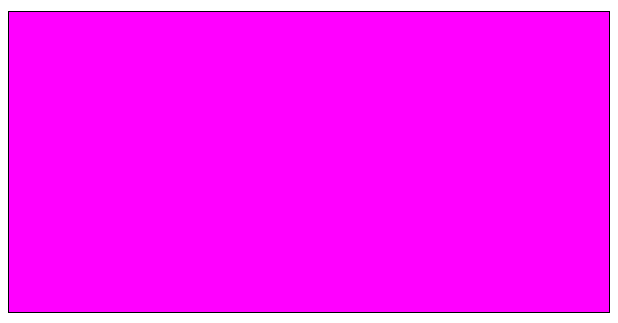
Drawing on Canvas
Here's an example of how you can draw a simple rectangle on the Canvas:
const canvas = document.getElementById('myCanvas');
const ctx = canvas.getContext('2d');
// Set fill color
ctx.fillStyle = 'red';
// Draw a filled rectangle
ctx.fillRect(50, 50, 100, 50);
In this example, we first obtain the Canvas element and its rendering context (ctx
). We then set the fill color to red using fillStyle
and draw a filled rectangle at position (50,50) with a width of 100 and a height of 50 using fillRect()
.
Complete Code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset=UTF-8>
<meta name=viewport content="width=device-width, initial-scale=1.0">
<title>Canvas Demo</title>
<style>
canvas {
border: 1px solid black;
}
</style>
</head>
<body>
<canvas id="myCanvas" width=400 height=200></canvas>
<script>
// JavaScript code will go here
const canvas = document.getElementById('myCanvas');
const ctx = canvas.getContext('2d');
// Set fill color
ctx.fillStyle = 'red';
// Draw a filled rectangle
ctx.fillRect(50, 50, 100, 50);
</script>
</body>
</html>
Output
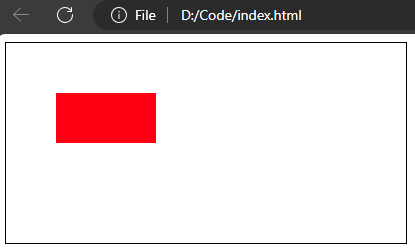
Leave a comment
Your email address will not be published. Required fields are marked *