Overview
Routing is a fundamental concept in modern web development, enabling the creation of single-page application (SPAs) with smooth navigation and user experiences. Angular provides a powerful routing module that simplifies the process of building complex navigational structures within your application. Angular provides a robust routing module, RouterModule
, that simplifies the process of implementing routing within your Angular application. In this comprehensive guide, we will explore routing in Angular from the basics to advanced techniques, covering everything you need to know to implement routing in your Angular applications. We will also explore the Angular RouterModule
from the ground up.
What is Angular Routing?
Angular Routing is a mechanism that allows you to navigate between different parts of a single-page application (SPA) while maintaining a consistent user interface. It enables developers to map URLs to specific views or components, making the application feel like traditional multi-page website.
In Angular, Routing is handled by the Angular Router Module (@angular/router
module).
Why Use Routing in Angular?
- Improved User Experience: Routing provides a seamless way for users to navigate between different sections or pages of an application.
- Modularization: It promotes modularity by dividing the application into distinct views, each associated with a specific route.
- Bookmarking and Sharing: With routing, users can bookmark or share specific URLs, making it easier to return to specific sections of the application.
What is RouterModule?
The RouterModule
is a key module in Angular's routing framework that provides the tools and infrastructure for setting up and managing routes within your application. It allows you to define routes, configure navigation, protect routes with guards, and handle route-related events seamlessly.
Getting Started with Angular Routing
Setting Up a New Angular Project
Before diving into routing with Angular Routing, you must create an Angular project. You can use the Angular CLI to do this:
ng new my-angular-app
Importing RouterModule
To utilize RouterModule, you need to import it into your Angular application's module. Import it from @angular/router
and include it in your module's imports
array:
import { RouterModule } from '@angular/router';
const routes: Routes = [];
// we define routes inside this array as array of objects.
@NgModule({
imports: [RouterModule.forRoot(routes)],
// ...
})
export class AppModule {}
Defining Routes
Creating a Route Configuration
Routes in Angular are defined as an array of route objects. Each route object maps a URL path to a component. Here's an example of defining some routes:
const routes: Routes = [
{ path: '', component: HomeComponent },
{ path: 'about', component: AboutComponent },
{ path: 'contact', component: ContactComponent },
];
Configuring Routes
Routes can be further configures with options such as redirectTo
and pathMatch
to handle default routes or empty paths:
const routes: Routes = [
{ path: '', redirectTo: '/home', pathMatch: 'full' },
// ...
];
Route Parameters and Wildcards
Wild Card Route: A wild card route is that route which matches every route path. In angular, the wild card route is specified using **
signs.
Note: A wild card route must be specified at the end of all the defined routes.
Angular supports dynamic routes with parameters and wildcards, allowing you to capture and use values from the URL:
**
= It matches all the path that user entered in the address bar.
const routes: Routes = [
{ path: 'products/:id', component: ProductDetailComponent },
{ path: 'search/:query', component: SearchResultsComponent },
{ path: '**', component: PageNotFoundComponent }, // Wildcard route
];
Router Outlets
The Role of Router Outlets
Router outlets are placeholders within your application's templates where routed views are displayed. They serve as entry points for route components:
<router-outlet></router-outlet>
Let's create a simple angular app using routing:
Step 1: Create a New Angular Project
Execute the below command to create an angular project:
ng new angular-routing-app
Follow the prompts to configure your project settings.

Step 2: Create Components
In this example, we will create three components: HomeComponent, AboutComponent, and ContactComponent.
ng generate component home
ng generate component about
ng generate component contact
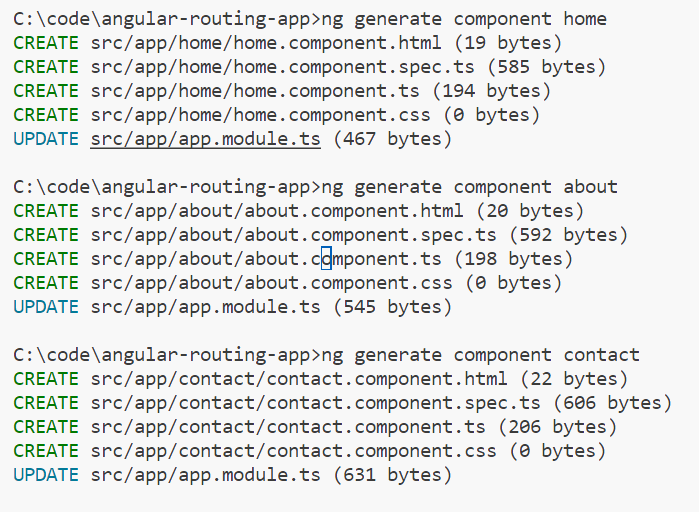
Step 3: Configure Routes
Open the src/app/app-routing.module.ts
file and configure your routes. Here's an example:
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
import { HomeComponent } from './home/home.component';
import { AboutComponent } from './about/about.component';
import { ContactComponent } from './contact/contact.component';
const routes: Routes = [
{ path: '', component: HomeComponent },
{ path: 'about', component: AboutComponent },
{ path: 'contact', component: ContactComponent },
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
Step 4: Add Router Outlet
In your src/app/app.component.html
file, add a <router-outlet></router-outlet>
tag where you want the routed components to be displayed:
<nav>
<a routerLink="/" routerLinkActive="active">Home</a>
<a routerLink="/about" routerLinkActive="active">About</a>
<a routerLink="/contact" routerLinkActive="active">Contact</a>
</nav>
<router-outlet></router-outlet>
Step 5: Start the Development Server
Run the development server to see your application in action:
ng serve
You can now access your app in your web browser at http://localhost:4200
.
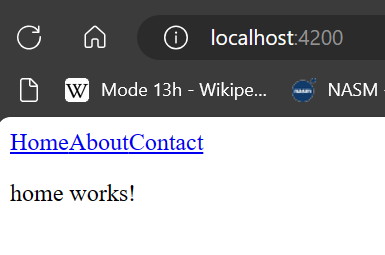
This simple Angular app with routing allows you to navigate between the Home, About, and Contact components by clicking on the navigation links.
Leave a comment
Your email address will not be published. Required fields are marked *