The Basics: Drawing a Line
To draw a line on an HTML5 Canvas, we utilize four essential Canvas API methods:
- Begin the Path: We start by telling the browser that we are about to draw a new path using the
beginPath()
method. - Move to the Starting Point: Next, we use the
moveTo(x, y)
method to position our drawing cursor at the starting point of the line. - Draw the Line: We then use the
LineTo(x, y)
method to define the ending point of the line and draw it by connecting the starting and ending points. - Make the Line Visible: Finally, we use the
stroke()
method to make the line visible on the canvas.
Example: The following code draws a line on canvas:
<!DOCTYPE HTML>
<html>
<body>
<canvas id="myCanvas" width=500 height=200></canvas>
<script>
let canvas = document.getElementById('myCanvas');
let context = canvas.getContext('2d');
// Draw a line
context.beginPath();
context.moveTo(100, 150);
context.lineTo(450, 50);
context.stroke();
</script>
</body>
</html>
Output:
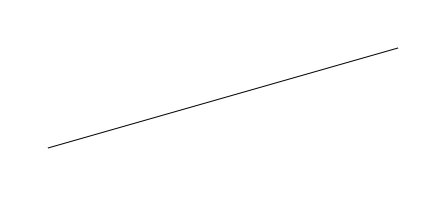
Customizing Your Lines
Now that we have mastered the basics, let's explore how we can customize our lines to make them stand out.
Line Width:
To change the thickness of our lines, we can use the lineWidth
property. This property controls the width of the lines in pixels. Here's how we can do, add the below line before stroke()
function:
context.lineWidth = 15; // Set line width to 15 pixels
Complete Example:
<!DOCTYPE HTML>
<html>
<body>
<canvas id="myCanvas" width=500 height=200></canvas>
<script>
let canvas = document.getElementById('myCanvas');
let context = canvas.getContext('2d');
// Draw a line
context.beginPath();
context.moveTo(100, 150);
context.lineTo(450, 50);
context.lineWidth = 15; // Set line width to 15 pixels
context.stroke();
</script>
</body>
</html>
Output:
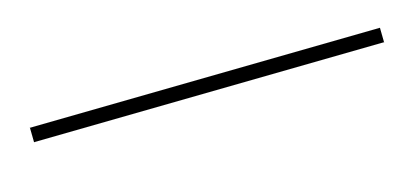
Line Color
We can also change the color of our lines using the strokeStyle
property. This property accepts color values in various formats, such as color names, hex values, or RGB values. Here how we can do it, use the below line:
context.strokeStyle = '#ff0000'; // Set line color to red
Complete Example:
<!DOCTYPE HTML>
<html>
<body>
<canvas id="myCanvas" width=500 height=200></canvas>
<script>
let canvas = document.getElementById('myCanvas');
let context = canvas.getContext('2d');
// Draw a line
context.beginPath();
context.moveTo(100, 150);
context.lineTo(450, 50);
context.lineWidth = 15; // Set line width to 15 pixels
context.strokeStyle = '#00ff00'; // Set line color to red
context.stroke();
</script>
</body>
</html>
Output:
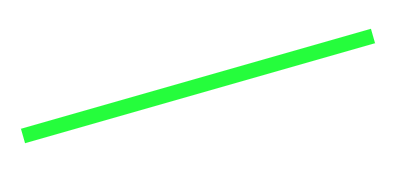
Exploring Line Cap Styles
Canvas lines can have different cap styles, which determine how the ends of the lines are rendered. The available cap styles are:
- butt: The default cap style, where lines end abruptly.
- round: Lines end with a rounded cap.
- square: Lines end with a square cap.
We can set this line cap style using the lineCap
property. Here's how to do it:
context.lineCap = 'round'; // Set line cap style to round
Complete Example:
<!DOCTYPE HTML>
<html>
<body>
<canvas id="myCanvas" width=500 height=200></canvas>
<script>
let canvas = document.getElementById('myCanvas');
let context = canvas.getContext('2d');
// butt line cap (top line)
context.beginPath();
context.moveTo(200, canvas.height / 2 - 50);
context.lineTo(canvas.width - 200, canvas.height / 2 - 50);
context.lineWidth = 20;
context.strokeStyle = 'red';
context.lineCap = 'butt';
context.stroke();
// round line cap (middle line)
context.beginPath();
context.moveTo(200, canvas.height / 2);
context.lineTo(canvas.width - 200, canvas.height / 2);
context.lineWidth = 20;
context.strokeStyle = 'black';
context.lineCap = 'round';
context.stroke();
// square line cap (bottom line)
context.beginPath();
context.moveTo(200, canvas.height / 2 + 50);
context.lineTo(canvas.width - 200, canvas.height / 2 + 50);
context.lineWidth = 20;
context.strokeStyle = '#EEEEEE';
context.lineCap = 'square';
context.stroke();
</script>
</body>
</html>
Output:
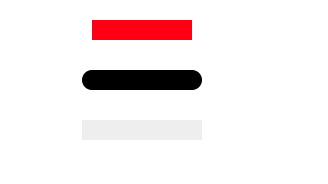