What is ngOnInit?
ngOnInit
is a lifecycle hook provided by Angular that is called once, after the component has been initialized. It is commonly used for performing initialization tasks such as fetching data from a server, initializing state variables, or setting up subscriptions.
This hook is raised after
ngOnChanges
.
- This hook is fired only once i.e. during the first change detection cycle. After that, if the input property changes, this hook does not gets called. Only ngOnChanges gets called.
How Does ngOnInit Work?
When a component is instantiated, Angular automatically calls the ngOnInit
method on that component, if it exists. This makes it an ideal place to perform initialization tasks that are necessary before the component is rendered or used.
How to Use ngOnInit
In order to use ngOnInit, we need to implement OnInit
interface in the component class and include OnInit
from the @angular/core
.
import { OnInit } from '@angular/core';
export class ExampleComponent implements OnInit {
Now let's define the ngOnit Hook function into the previous example Code:
child-component.components.ts:
import { Component, Input, OnChanges, OnInit, SimpleChanges } from '@angular/core';
@Component({
selector: 'app-child-component',
templateUrl: './child-component.component.html',
styleUrls: ['./child-component.component.css']
})
export class ChildComponentComponent implements OnChanges, OnInit {
@Input() message: string = "";
constructor() {
console.log("Child Constructor called.");
}
ngOnChanges(changes: SimpleChanges) {
console.log("ngOnChanges");
console.log(changes);
}
ngOnInit(){
console.log("ngOnInit");
}
}
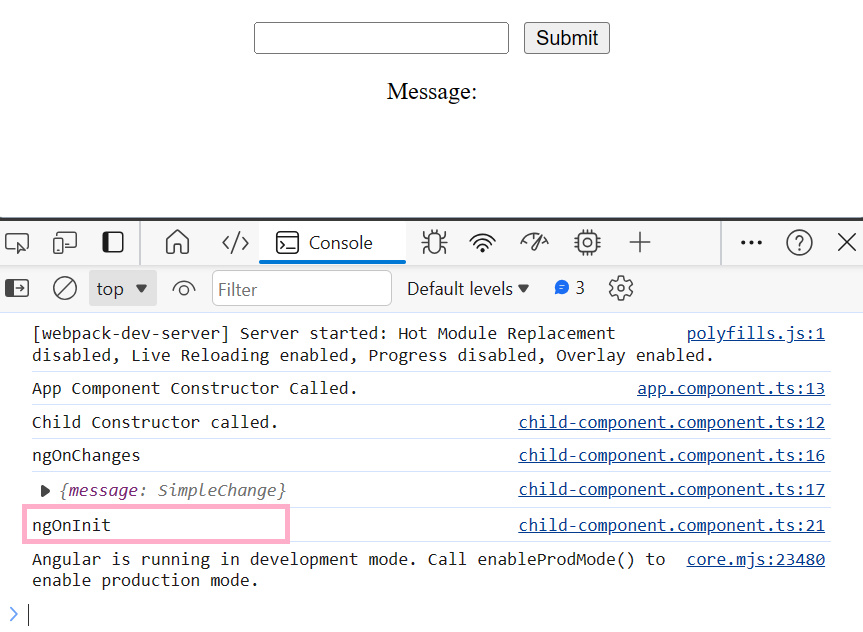
Notice from the console log picture above that ngOnInit
is called after ngOnChanges
initially.
Now, let's see what happens when we change the Input property message from the parent component.
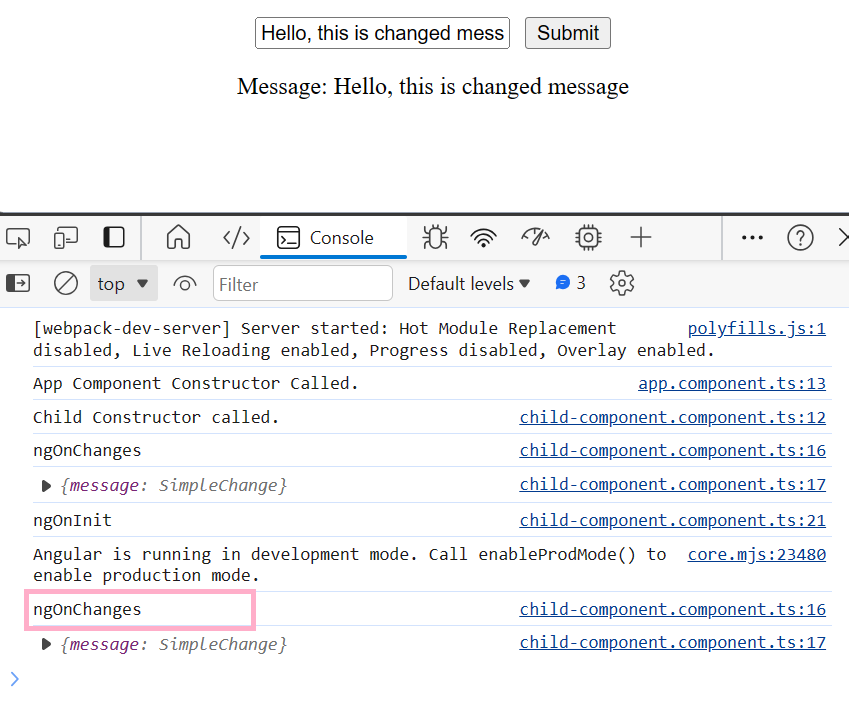
We observe that only ngOnChanges
get called not ngOnInit
. That's because ngOnInit
called only once during initialization only.
Leave a comment
Your email address will not be published. Required fields are marked *