Overview
To ensure project organization and maintainability, Angular projects come with a well-defined directory structure. In this article, we will dive deep into the Angular project directory structure, exploring each folder's purpose and the best practices for organizing your code.
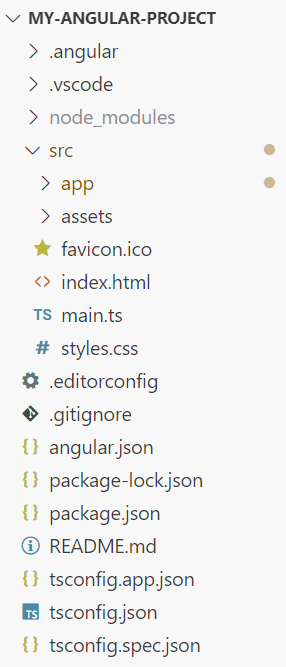
Angular Project Directory Structure
Here's an overview of the key directories and files in a typical Angular project:
1. src: This is the heart of your Angular application. It contains all your source code. Every component, service class, modules resides in here only. Whenever we create an angular project, by default the angular framework creates lots of files within the src folder which is shown in the image below.
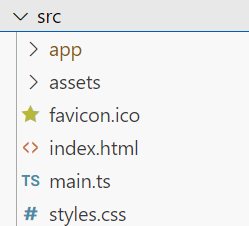
As you can see src folder contains many subfolders and files. Let us discuss the use and need of each subfolder and files.
- app: This is where you application-specific code resides. Contains the component files in which your application logic and data are defined. Have a look at the image shown below which shows the files which are by default created within the app folder when you create an angular application. As you can see, by default it creates one component (
app.component.ts
) and one module (app.module.ts
) and an angular application should have at least one component and one module.
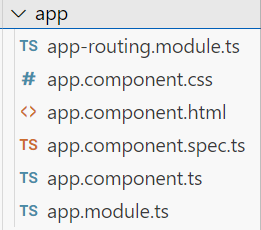
- components: Store your Angular components here.
- Example:
app-header
,product-list
,user-profile
.
- Example:
- services: Place your Angular services, which provide data and functionality to components.
- Example:
auth.service.ts
,product.service.ts
.
- Example:
- modules: Organize your application into feature modules.
- Example:
auth.module.ts
,product.module.ts
.
- Example:
- guards: Implement route guards for protecting routes.
- Example:
auth.guard.ts
.
- Example:
- assets: Store static assets like images, fonts, and JSON files.
- favicon.ico: An icon to use for this application in the bookmark bar.
- environments: Manage environment-specific configurations.
environment.ts
: Development environment.environment.prod.ts
: Production environment.
- styles.css: Define global styles and CSS preprocessors (e.g., SCSS) here.
- index.html: The main HTML file for your application. This HTML page is served when someone visits your site. The CLI automatically adds all JavaScript and CSS files when building your app.
- main.ts: The main entry point for your application. Compiles the application with the JIT compiler and bootstraps the applications's root module (AppModule) to run in the browser.
2. e2e: End-to-end (E2E) testing file using tools like Protractor.
3. node_modules: This folder contains the packages (folders of the packages) which are installed into your application when you created a project in angular. If your installed any third-party packages then also their folders are going to be stored within this node_modules folder.
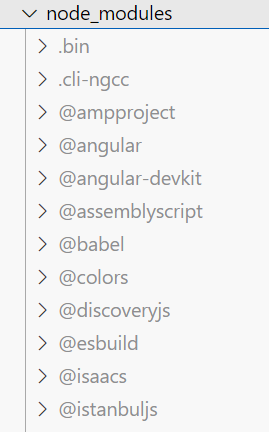
4. angular.json: Angular CLI configuration file for project settings.
5. tsconfig.json: TypeScript configuration for the project.
6. package.json: Lists project dependencies, scripts, and metadata.
7. package-lock.json: Lock file for package versions. Provides version information for all packages installed into node_modules by the npm client.
8. tslint.json: TypeScript linting rules for the project.
9. editorconfig: Configuration for code editors.