Overview
Node.js comes with a set of built-in core modules that extend its functionality. In this chapter, we will explore Node.js core modules, understand their purpose, and explain how to use them in your applications.
What are Node.js Core Modules?
Node.js core modules are a collection of built-in modules that provide essential functionalities to Node.js applications. They are included with Node.js installation, so your don't need to install them separately. These modules cover a wide range of tasks, from working with the file system to handling network communication.
Commonly Used Core Modules
Let's take closer look at some of the most commonly used core modules in Node.js:
fs
(File System)
The fs
module allows you to work with the file system, making it possible to read and write files, create directories, and manager file operations.
Example - Reading a File:
const fs = require('fs');
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) {
console.error(err);
return;
}
console.log(data);
});
console.log("The next line");
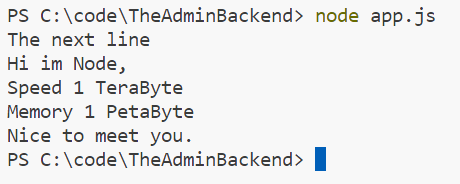
http
(HTTP Server and Client)
The http
module provides the foundation for creating HTTP servers and clients, making it possible to build web applications and APIs.
Example - Creating an HTTP Server:
const http = require('http');
const server = http.createServer((req, res) => {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end('Hello, World!\n');
});
server.listen(3000, () => {
console.log('Server is running on http://localhost:3000/');
});
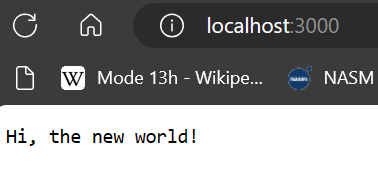
path
(File and Directory Paths)
The path
module simplifies working with file and directory paths, handling differences between operating systems.
Example - Joining Paths:
const path = require('path');
const fullPath = path.join(__dirname, 'files', 'example.txt');
console.log(fullPath);
os
(Operating System Information)
The os
module provides information about the operating system on which your Node.js application is running.
Example - Getting System Information:
const os = require('os');
console.log('Hostname:', os.hostname());
console.log('Platform:', os.platform());
console.log('Total Memory (in bytes):', os.totalmem());
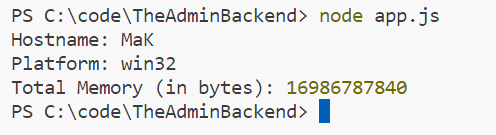
events
(Event Emitter)
The events
module allows you to create and handle custom events in your application. It's the foundation for building event-driven architectures.
Example - Creating an Event Emitter:
const EventEmitter = require('events');
class MyEmitter extends EventEmitter {}
const myEmitter = new MyEmitter();
myEmitter.on('customEvent', () => {
console.log('Custom event occurred.');
});
myEmitter.emit('customEvent');
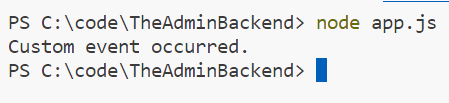
util
(Utilities)
The util
module provides utility functions that help with debugging, inheritance, and other common tasks.
Example - Using the util.promisify
Function:
const util = require('util');
const fs = require('fs');
const readFilePromised = util.promisify(fs.readFile);
readFilePromised('example.txt', 'utf8')
.then(data => {
console.log(data);
})
.catch(err => {
console.error(err);
});
Using Core Modules in Node.js
To use a core module in your Node.js application, you need to require it at the beginning of your code using the require
statement. For example:
const fs = require('fs');
Once you have required a core module, you can access its functions and classes and use them in your application.