In a computer, a stack is a type of data structure where items are added and then removed from the stack in reverse order. That is, the most recently added item is the very first one that is removed. This is often referred to as Last-In, First-Out (LIFO).
A stack is heavily used in programming for the storage of information during procedure or function calls.
Adding an item to a stack is referred to as a push or push operation. Removing an item from a stack is referred to as a pop or pop operation.
Stack Example
To demonstrate the general usage of the stack, given an array, a = {7, 19, 37},
Consider the operations:
push a[0]
push a[1]
push a[2]
Followed by the operations:
pop a[0]
pop a[1]
pop a[2]
The initial push will push the 7, followed by the 19, and finally the 37. Since the stack is last-in, first-out, the first item popped off the stack will be the last item pushed, or 37 in this example. The 37 is placed in the first element of the array (over-writing the 7). As this continues, the order of the array elements is reversed.
The following diagram shows the progress:
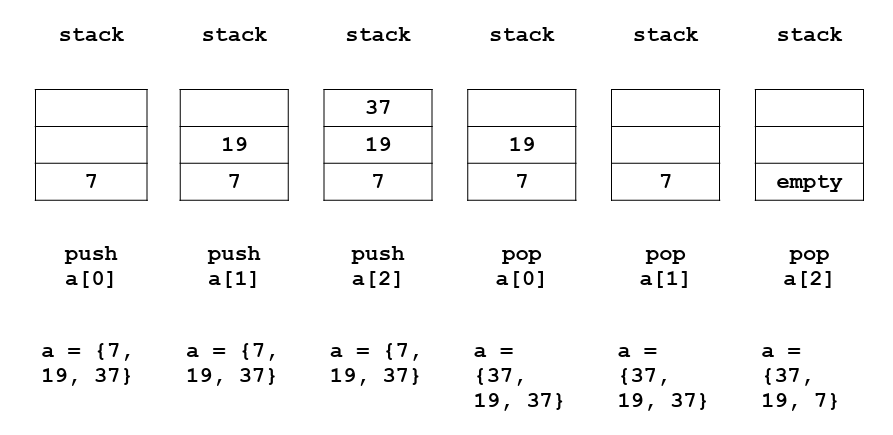
Leave a comment
Your email address will not be published. Required fields are marked *