Overview
Node.js is a powerful runtime environment for executing JavaScript on the server-side. It's an excellent choide for building web applications and APIs. In this article, we will guide through creating your first Node.js application from scratch, and we will explaining each step with code examples.
Prerequisites
Before you start, make sure you have Node.js and npm (Node Package Manager) installed on your system.
Step 1: Setting Up Your Project
First, create a project directory and navigate to it using your terminal or command prompt:
mkdir my-first-node-app
cd my-first-node-app
Step 2: Initializing a Node.js Project
To manage your project's dependencies and configuration, you should initialize a Node.js project by running the following command:
npm init
You will be prompted to answer several questions about your project. You can simply press Enter to accept the default values for most of them.
Step 3: Creating Your Entry Point
In Node.js, the entry point of your application is typically a JavaScript file. Create a file called app.js
in your project directory:
touch app.js
Now, open app.js
in your code editor. This is where you will write your first Node.js code.
Step 4: Writing Your First Node.js Code
Let's start with a simple Hello, World!
example. In app.js
, add the following code:
// Import the built-in 'http' module
const http = require('http');
// Create an HTTP server that responds with "Hello, World!" to all requests
const server = http.createServer((req, res) => {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end('Hello, World!\n');
});
// Listen on port 3000
server.listen(3000, () => {
console.log('Server is running on http://localhost:3000/');
});
This code does the following:
- It imports the built-in
http
module, which provides the functionality to create an HTTP server. - It creates an HTTP server that listens for incoming requests.
- The server responds with
Hello, World!
to all requests. - The server listens on port 3000, and when it starts, it logs a message to the console.
Step 5: Running Your Node.js Application
To run your Node.js application, execute the following command in your terminal:
node app.js
You should see the message Server is running on http://localhost:3000/
in your terminal.
Step 6: Testing Your Application
Open a web browser and visit http://localhost:3000/
. You should see Hello, World!
displayed in your browser.
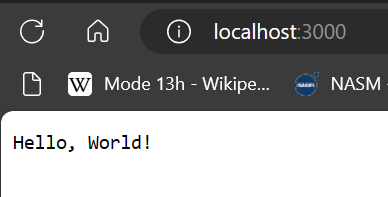