Overview
In Angular, the concept of template references, often referred to as template reference variables, is a fundamental building block for creating dynamic and interactive applications. This chapter will provide a comprehensive understanding of template references, their uses, practical examples, and how to leverage them to work with elements and components in your Angular templates.
Understanding Template References
Template references allow you to create a reference to an element, component, or directive in your Angular template. These references are declared using the #
symbol followed by a variable name. Once defined, you can access and manipulate the referenced element or component in your component's code.
Declaraing Template References
To declare a template reference, use the #
symbol followed by a variable name. Here's a basic example:
<input #inputRef type=text />
<button (click)="logInputValue(inputRef.value)">Log Value</button>
In the above code:
- #inputRef is a template reference variable associated with the
<input>
element. - The
logInputValue
method is called when the button is clicked and it passes the value of the input element.
Accessing Elements
Template references are frequently used to access and manipulate DOM elements. For example, you can access the input element's vale and change its properties or styles:
import { Component } from '@angular/core';
@Component({
selector: 'app-example',
template: `
<input #inputRef type=text />
<button (click)="logInputValue(inputRef.value)">Log Value</button>
`,
})
export class ExampleComponent {
logInputValue(value: string) {
console.log(`Input value: ${value}`);
}
}
In the component code:
inputRef
is used to reference the input element.- The
logInputValue
method accesses the value of the input element.
Using Template References with Components
Template references are not limited to DOM elements. You can also use them to reference Angular Components. For instance, if you have a child component within your parent component, you can access its methods and properties using a template reference.
Step 1: Create the Child Component
First, create a child component. In this example, we will create a simple child component that has a method to display a message. Here's the code for the child component:
child.component.ts:
import { Component } from '@angular/core';
@Component({
selector: 'app-child',
template: `
<div>
<p>Child Component</p>
<button (click)="displayMessage()">Display Message</button>
</div>
`,
})
export class ChildComponent {
displayMessage() {
alert('Hello from the child component!');
}
}
Step 2: Create the Parent Component
Now, create a parent component that includes the child component and uses a template reference to access the child's methods. Here's the code for the parent component:
parent.component.ts:
import { Component } from '@angular/core';
@Component({
selector: 'app-parent',
template: `
<div>
<h1>Parent Component</h1>
<app-child #childRef></app-child>
<button (click)="callChildMethod(childRef)">Call Child Method</button>
</div>
`,
})
export class ParentComponent {
callChildMethod(child: ChildComponent) {
child.displayMessage();
}
}
In the parent component's template:
- We include the child component using
<app-child #childRef></app-child>
. Here, we declare a template reference variable#childRef
for the child component. - We have a button that, when clicked, calls the
callChildMethod
method, passing thechildRef
as an argument.
Step 3: Displaying the Result
When you run the application and click the “Call Child Method” button in the parent component, it will call the displayMessage
method of the child component, displaying the message “Hello from the child component!” in an alert.
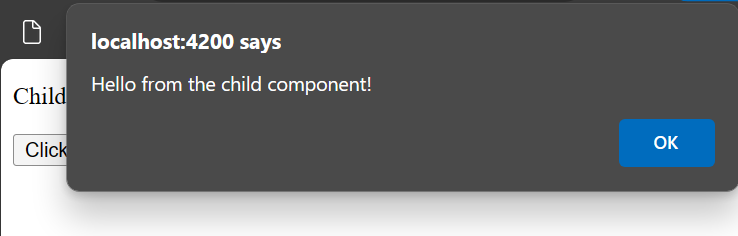