Understanding the arc() Method
The arc()
method
- Center Point(x, y): The coordinates of the center point of the circle.
- Radius: The radius of the circle.
- Starting Angle: The angle at which the arc begins, in radians.
- Ending Angle: The angle at which the arc ends, in radians.
- Direction: Specifies the drawing direction, either clockwise or anticlockwise.
Here's the syntax of the arc()
method:
context.arc(x, y, radius, startAngle, endAngle, counterclockwise);
Example: Drawing an Arc
<!DOCTYPE HTML>
<html>
<body>
<canvas id="myCanvas" width=500 height=250></canvas>
<script>
var canvas = document.getElementById('myCanvas');
var context = canvas.getContext('2d');
var x = canvas.width / 2;
var y = canvas.height / 2;
var radius = 75;
var startAngle = 0.5 * Math.PI;
var endAngle = 1.5 * Math.PI;
var counterClockwise = false;
// Draw an arc
context.beginPath();
context.arc(x, y, radius, startAngle, endAngle, counterClockwise);
context.lineWidth = 5;
context.stroke();
</script>
</body>
</html>
Output:
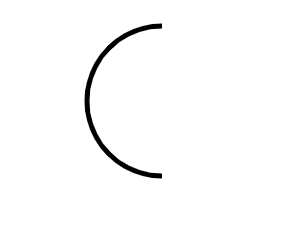
Creating Different Shapes with arc()
Now, let's explore how we can use the arc()
method to create various shapes on the canvas:
Drawing a Circle
To draw a circle, we set the starting angle to 0 and the ending angle to 2 * Math.PI
:
context.beginPath();
context.arc(centerX, centerY, radius, 0, 2 * Math.PI, false);
Complete Example:
<!DOCTYPE HTML>
<html>
<body>
<canvas id="myCanvas" width=500 height=200></canvas>
<script>
let canvas = document.getElementById('myCanvas');
let context = canvas.getContext('2d');
let centerX = canvas.width / 2;
let centerY = canvas.height / 2;
let radius = 70;
context.beginPath();
context.arc(centerX, centerY, radius, 0, 2 * Math.PI, false);
context.fillStyle = 'gray';
context.fill();
context.lineWidth = 5;
context.strokeStyle = 'violet';
context.stroke();
</script>
</body>
</html>
Output:
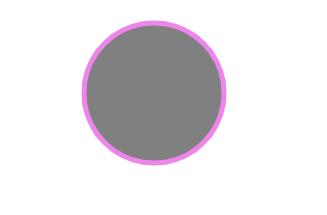
Creating a Semicircle
For a semicircle, we define the ending angle as startAngle + Math.PI
:
context.beginPath();
context.arc(200, 75, 70, 0, Math.PI, false);
Complete Example:
<!DOCTYPE HTML>
<html>
<body>
<canvas id="myCanvas" width=500 height=200></canvas>
<script>
let canvas = document.getElementById('myCanvas');
let context = canvas.getContext('2d');
let centerX = canvas.width / 2;
let centerY = canvas.height / 2;
let radius = 70;
context.beginPath();
// draw the arc in the fourth and third quadrant.
// meaning start at angle 0 anticlockwise and end at angle 180.
context.arc(centerX, centerY, 70, 0, Math.PI, false);
context.fillStyle = 'gray';
context.fill();
context.lineWidth = 5;
context.strokeStyle = 'violet';
context.stroke();
</script>
</body>
</html>
Output:
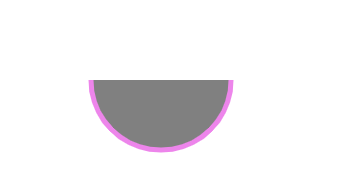
Drawing Rounded Corners
To create rounded corners, we can use the arcTo()
method, defining control points, ending points, and a radius:
context.beginPath();
context.arcTo(rectX + rectWidth, rectY, rectX + rectWidth, rectY + cornerRadius, cornerRadius);
Complete Example:
<!DOCTYPE HTML>
<html>
<body>
<canvas id="myCanvas" width=500 height=200></canvas>
<script>
let canvas = document.getElementById('myCanvas');
let context = canvas.getContext('2d');
let rectWidth = 200;
let rectHeight = 100;
let rectX = 189;
let rectY = 50;
let cornerRadius = 50;
context.beginPath();
context.moveTo(rectX, rectY);
context.lineTo(rectX + rectWidth - cornerRadius, rectY);
context.arcTo(rectX + rectWidth, rectY, rectX + rectWidth, rectY + cornerRadius, cornerRadius);
context.lineTo(rectX + rectWidth, rectY + rectHeight);
context.lineWidth = 5;
context.stroke();
</script>
</body>
</html>
Output:
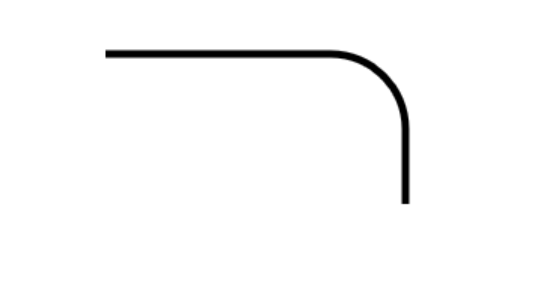